Two Pass Assembler
A two-pass assembler performs two sequential scans over the source code:
Pass 1: symbols and literals are defined
Pass 2: object program is generated
Parsing: moving in program lines to pull out op-codes and operands
Data Structures:
- Location counter (LC): points to the next location where the code will be placed
- Op-code translation table: contains symbolic instructions, their lengths and their op-codes (or subroutine to use for translation)
- Symbol table (ST): contains labels and their values
- String storage buffer (SSB): contains ASCII characters for the strings
- Forward references table (FRT): contains pointer to the string in SSB and offset where its value will be inserted in the object code
assembly machine
language Pass1 Pass 2 language
program Symbol table program
Forward references table
String storage buffer
Partially configured object file
Figure 2. A simple two pass assembler.
Example 1: Decrement number 5 by 1 until it is equal to zero.
assembly language memory object code
program address in memory
-----------------------
START 0100H
LDA #5 0100 01
0101 00
0102 05
LOOP: SUB #1 0103 1D
0104 00
0105 01
COMP #0 0106 29
0107 00
0108 00
JGT LOOP 0109 34
010A 01 placed in Pass 1
010B 03
RSUB 010C 4C
010D 00
010E 00
END
Op-code Table
Mnemonic
| Addressing mode
| Opcode
| LDA
| immediate
|
| SUB
| immediate
| 1D
| COMP
| immediate
|
| LDX
| immediate
|
| ADD
| indexed
|
| TIX
| direct
| 2C
| JLT
| direct
|
| JGT
| direct
|
| RSUB
| implied
| 4C
|
Symbol Table
Example 2: Sum 6 elements of a list which starts at location 200.
assembly language memory object code
program address in memory
-----------------------
START 0100H
LDA #0 0100 01
0101 00
0102 00
LDX #0 0103 05
0104 00
0105 00
LOOP: ADD LIST, X 0106 18
0107 01 placed in Pass 2
0108 12
TIX COUNT 0109 2C
010A 01 placed in Pass 2
010B 15
JLT LOOP 010C 38
010D 01 placed in Pass 1
010E 06
RSUB 010F 4C
0110 00
0111 00
LIST: WORD 200 0112 00
0113 02
0114 00
COUNT: WORD 6 0115 00
0116 00
0117 06
END
Symbol Table
Symbol
| Address
| LOOP
|
| LIST
|
| COUNT
|
|
Forward References Table
Offset
| SSB pointer for the symbol
|
| DC00
| 000A
| DC05
|
SSB
DC00
| 4CH
|
| DC01
| 49H
| ASCII for L,I,S,T
| DC02
| 53H
|
| DC03
| 54H
|
| DC04
| 5EH
| ASCII for separation character
| DC05
|
|
| Pass1
· All symbols are identified and put in ST
· All op-codes are translated
· Missing symbol values are marked

LC = origin

Read next statement


Parse the statement

Y
Comment
N

“END” Y Pass 2
N


N pseudo-op Y what
kind?
N
Label EQU WORD/ RESW/RESB
BYTE
Y
N Label N Label
Enter label in ST Enter label in ST
Y Y
Enter label in ST Enter label in ST
Call translator
Place constant in
machine code
Advance LC by the
number of bytes specified
Advance LC in the pseudo-op

Figure 3. First pass of a two-pass assembler.
Translator Routine

Find opcode and the number of bytes
in Op-code Table

Write opcode in machine code


Write the data or address that is known
at this time in machine code
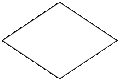
more
information Y
will be needed Set up an entry in
in Pass 2 ? Forward References Table
N

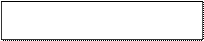
Advance LC by the number of bytes
in op-code table

return
Figure 4. Flowchart of a translator routine
Pass 2
- Fills addresses and data that was unknown during Pass 1.
Pass 1
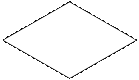
More lines in N
Forward References Done
Table
Y

Get the next line

Retrieve the name of the symbol from SSB

Get the value of the symbol from ST
Compute the location in memory
where this value will be placed
(starting address + offset)

Place the symbol value at this location
Figure 5. Second pass of a two-pass assembler.
Date: 2015-01-11; view: 1465
|